Okay, so today I messed around with something called a “movie screen canvas.” Sounds fancy, right? Well, it wasn’t as complicated as it sounds, but it did take me a bit of time to figure out. Basically, I wanted to create this cool effect where a video plays like it’s on a movie screen.
First, I grabbed an HTML file and threw in a video element. I made sure to include the “controls” attribute so I could actually play, pause, and all that good stuff. I also picked a video from my own computer, nothing special, just something to test this out. After I set up the basic HTML, I saved the file and double-clicked it to open it in my browser. You know, the usual routine when you’re testing web stuff.
Next up, I made a canvas element. Now, this is where the “movie screen” part comes in. The canvas is like a blank slate where you can draw stuff using JavaScript. And, in this case, I was going to “draw” the video frames onto it, which is kind of like projecting the video onto the canvas.
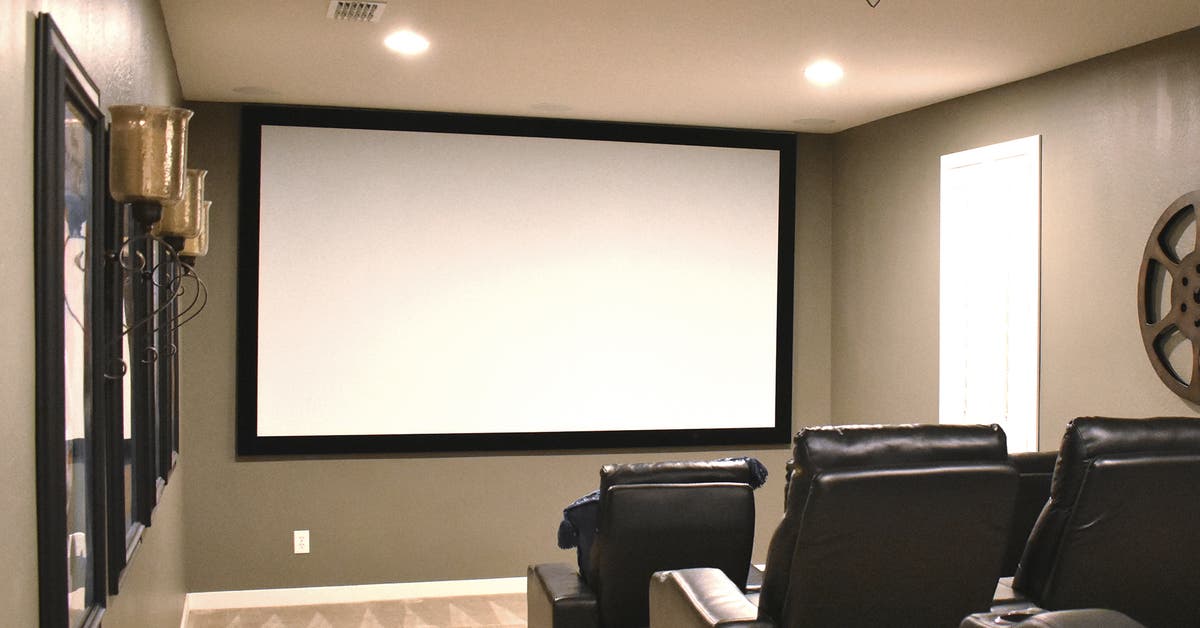
So, I fired up some JavaScript. Initially, I had to grab the video and canvas elements from the HTML. I did that using the usual “getElementById” method. Then I had to get the canvas’s drawing context. In my case, I used 2d as context type. This is basically like getting your paintbrush and palette ready. I also got the canvas’s width and height.
Now came the tricky part. I needed a way to constantly update the canvas with the video frames. For that, I used this thing called “requestAnimationFrame”. Think of it like a loop that keeps running and updating the canvas as long as the video is playing. And inside this loop, I used the “drawImage” method to actually put the video frame onto the canvas. I even set some drawing styles for fun. And once the video ends, I just cleared the canvas.
After all that coding, I went back to my browser and refreshed the page. And voila! The video was playing on the canvas, just like I wanted. It wasn’t exactly IMAX quality, but it was still pretty cool to see it working. I did some testing, including pausing and playing. Everything went well, and I was quite happy about the final result.
Here’s a little breakdown of what I did:
- Created an HTML file with a video and a canvas element.
- Got the video and canvas elements in JavaScript.
- Set up the canvas’s 2D drawing context.
- Used “requestAnimationFrame” to create a loop that updates the canvas.
- Used “drawImage” to draw the video frames onto the canvas.
- Cleared the canvas when the video ended.
All in all, it was a fun little experiment. It’s always cool to learn something new and see it come to life on your screen. Maybe next time I’ll try adding some fancy effects or something, but for now, I’m pretty happy with my simple movie screen canvas.